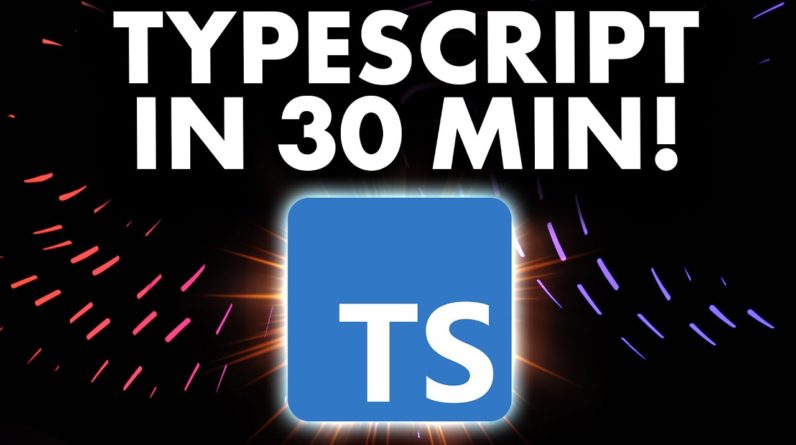
in this video I'll teach you everything you need to know about typescript as fast as possible so let's start with what typescript is now typescript is a superset of JavaScript which means it extends the capabilities of JavaScript by adding optional static types developed and maintained by Microsoft it was first released in 2012. now typescript helps you write more robust and maintainable code thanks to its type checking features it catches potential errors during development rather than at runtime which saves you tons of time and effort now don't worry about browser compatibility as typescript compiles down to plain JavaScript ensuring that your code runs smoothly across different platforms typescript is well suited for large-scale applications or when working with teams as it enhances the code readability and maintainability to sum it up typescript brings the benefits of static typing to JavaScript making it a powerful Ally for developing modern web applications now let's dive into setting up typescript and writing our first typescript code alright so I'm going to get into setting up and installing typescript but I did want to mention that if you're looking to get better at programming I do have a programming course called programmingexpert.io that you guys can check out from the link in the description and if you're at all interested in blockchain and web3 technology and becoming a developer in that space then check out my other course blockchain expert again from the link in the description now let's set up typescript so the first thing we need to do to set this up is download node.js I imagine many of you already have this installed if you don't go to the node.js website install node.js once you have that installed we're going to open up some kind of directory that will do this project in and we're going to install typescript globally just so we have access to the typescript command from any directory so I'm going to type npm install Dash G and then typescript this only works when you have node.js installed now this will install typescript globally for us I'll be back once it is finished alright so that command finished pretty quickly if we want to check if this is working we can type the command tsc-v and that should give us the version of typescript that we're using if you see some output here then that means that installation was successful now that we've done that I'm going to create a new directory here or a new npm package sorry and then we're going to set up typescript by creating a tsconfig.json file so I'm going to type npm init Dash y this is going to create a package Json file for me in this directory so now I have an npm project and now I'm going to create a new file this is TS config dot Json this is where we're going to put configuration for typescript so I'm just going to paste in here a fairly basic configuration and then we'll go through the options so you can see that we have a Json object we have our compiler options and then we're specifying the target is es5 the type of module is common.js we're using strict mode the output directory is dis-t now this is the directory that our compiled typescript code will go inside of so essentially it's going to transpile our typescript code into JavaScript and that will then live in the dist folder and then I have skip libcheck which which is just going to skip checking any of our node modules or libraries so we don't have to actually go through the process of doing all the type checking on them you can disable this if you want but I usually just enable it and then I have include and this is specifying what files I want to include in my kind of typescript project here so in this case I'm including anything inside of SRC this here means that it can be any subdirectory of SRC and then I'm specifying that it needs to end in dot TS so this is a basic configuration for typescript now notice here if I hover over this that I'm getting an error and saying no inputs were found in the config file and that's because we have not created this SRC directory so what I'm going to do is create a directory here called SRC this is where typescript expects that our files are going to live and then I'm going to create a file here called index.ts and note that TS is the typescript extension and then you get the little TS logo here if you're inside of Visual Studio code alright so now inside of our TS file I'm just going to write some very basic typescript and then show you how the transpiling or compiling whatever you want to refer to it as works so I'm going to say let X number equal three okay so some basic typescript code where I'm specifying the type of this variable and now I want to show you that if I want to compile this code into JavaScript all I need to do is run from the directory where my ts config file is the TSC command this is kind of the Alias for building so if I do this and hit enter it's going to build our project into this DISD folder and you can see that we have our index.js where we have the same thing that we had here except now kind of converted into regular JavaScript where we don't have this type annotation all right so now that we've set up typescript let's have a look at some of the types that you can use within typescript so let's create some variables let's say let X colon and we can start with a number equal three just like we had before now note here that this is the way that you annotate types you put a colon and then you specify the type that this variable is going to be in this case I've made it equal to three I also could just Define the variable like this and then I could assign it later on so like x equals 2.
Now if I try to do something like x equals hello you're going to see that I get an error here it's telling me the type string is not assignable to type number there you go you've already seen a massive benefit of typescript in regular JavaScript you wouldn't get this type of error because there would be nothing specifying that X needs to be a number so very helpful for larger development projects regardless that's one of the most common types another type you can use here is a string fairly straightforward you can also use a Boolean values that's going to be true or false and you can use the any value now the any value is essentially skipping creating a type for a variable or for whatever it is that you're attempting to type this means that you can have anything inside of the X variable so really it's kind of saying no type so you put any I don't know if that makes sense or not but I can do something like x equals 4 x equals hello and typescript will not get mad at me because it has the type of any now one thing to note here is that you don't need need to manually specify the types for your variables they can actually be implied that's the same with your functions and with really anything else in typescript so if I do something like const hello is equal to hello world and then I go down here and I try to say something like hello minus eight you're going to see that I get an error and that's because it knows that the type of hello is a string right so the left hand side of an arithmetic operation must be of type any number or big int and since this is not of that type it's not going to work if I hover over this uh it actually doesn't tell me that it's type string but it does know implicitly that it is the type string because that's what I assigned to this variable alright so continuing we have the type of unknown so I could do something like let y unknown like that now the difference with unknown and any is that if you attempt to use the unknown type you're going to have to type cast this or convert it into a different type to be able to use it properly whereas with any it essentially kind of Skips doing any of the type checking we'll have a look at this later on but understand that any and unknown are different any is just going to kind of allow you to skip all of the type checking whereas unknown is going to now force you to do all kinds of type casting and checking to be able to use this variable properly alright so next we have the type of void now void is used when you want to specify the function is not going to return any value so let's say we create a standard function here I'm going to say function X and then we'll just put our parentheses here now if I wanted to annotate this function which I don't need to do but I can do I can put a colon here and then put void and this now indicates this function is not returning any type of value now same thing if I wanted to do function parameters here I could do something like let's say num one and this is a number and num2 this is a number as well now I've kind of typed this function and I know that I'm accepting two numbers for my parameters and I am returning nothing okay so that is the void type UL obviously could put a different type here like number and then that would mean that you're going to return a number however I have to actually return a number otherwise this is going to get mad at me so I'm returning num1 plus num2 and now I don't get any errors all right the next type that we have is no so of course I can do something like VAR uh vowel no is equal to no that is perfectly fine that is the null type continuing we have the undefined type so if I say Val undefined then obviously I can make this equal to an undefined value sometimes that's useful and we'll look at some more complicated types where you might use undefined all right next we have the array type so I can of course do something like VAR nums and then say number like this and then put my array symbol here or my two brackets then make this equal to an array of different numbers that is a valid type we can then have a two-dimensional array if we wanted to do that so now we're going to have to have an array inside of an array for this to be a valid type now Beyond array we actually have another type known as a tuple or a tuple however you want to refer to it as so let's say I have kind of an interesting object here let's just call this obj where what I actually want to have is a string and a number so what I can do is specify the type like this this is now a tuple type and that means that I have to have an array that has exactly two values one of which is string and one of which is a number so I'll say hello and two like that and now this is valid that's how you specify a tuple continuing we have the object type so I can put object like this and now it's expecting that we have some kind of object here there you go those are the core types in typescript now let's have a look at how we can create custom types which are probably the more useful things that you're going to be using quite a bit in typescript and some more examples of just typing our objects and using these different typescript features all right so moving on now to custom types which is probably why most of you are watching this video in typescript if you want to create a custom type use the type keyword and then you set the name for the type so I'll do a type like animal inside of here I now specify all of the properties that I want something of this type to have so I can do something like name and this can be a string maybe we have age this is going to be a number maybe we have the colors of this animal and this will be a string array and then maybe we have some optional Fields like let's say legs and this can be a number now notice I'm using this question mark here to specify that this is an optional value so we might have it or we might not have it and that means implicitly typescript is going to say that legs is a number or an undefined type because we might not have this property so it could be undefined or if we do have it it's going to be a number and I'll show you how we handle that in just a minute okay so this is a very basic type now of course we can continue and create different types and typically it is a good idea to put all of your types inside of a types file so you might have a types.ts file inside of here you might say something like export type call this maybe type one and then you would specify the type and then you would import this in the files that you needed it obviously that can get a bit messy if you have tons of different types you might want to separate them by subdirectory or where they're being used but just something to note that a lot of people will kind of dump all of their types inside of one file like types.ts all right so let me just create an animal here I'm going to say const dog is equal to and then I'm going to say name is Tim I will say the age is 10 and the colors are Brown and black okay so now I've created something that does actually adhere to this animal type but you'll notice that if I hover over it it's not specifying that this is type animal and that's because I've not annotated it as an animal so I don't need to do that to be able to use this like an animal object but sometimes it's going to be helpful for you to manually animate annotate this story so that you know that it's of this type so I'm saying dog now it's telling me it's a type animal so that's one way to do that another way to do this is to type as and then animal this is essentially a type cast or conversion where what we're doing is we're taking this type and we're telling uh typescript to treat it as the animal type so now if I hover over this same thing we're able to uh implicitly kind of Define that this is of type animal now let's just remove this just so I can show you that we can use this like an animal so I'm going to create a function here let's say const print animal we'll make this equal to an arrow function and we'll just take in an animal that is of type animal and then what I can do is say console.log and I will print the animal.name and the animal.h now you'll notice that if I pass this to print animal this is fine I don't get any type errors here and that's because again this can be treated as the animal type because it has the correct Fields if I were to comment out one of these fields here and get rid of the name then notice that I get an error because it says argument of type h colors is not assignable to parameter of animal uh property name is missing in this type and same thing if I try to now annotate this as an animal we're still going to get this error because we don't have name defined which is a property that we need however even though we are not including legs everything is fine now let me quickly show you what happens if we try to access this legs property so if I go here and I say animal dot legs it's fine and the reason why we can do this in the print statement is because it's either going to print undefined if it's not defined or the number of legs but if I tried to do something like const X is equal to animal.legs minus five notice I'm going to get an error here because this could be an undefined value so if this is the case what you can do and I don't recommend this but you can do this is you can use the bang operator and what this will do is make it so that typescript treats this as if it's a defined value now the issue with doing this is that you can sometimes have an undefined value that you attempt to use a bang on and that means we're now going to have undefined minus five and when we have that that's going to raise an exception for us at runtime which we don't want to occur so instead of using this bang what I'll typically do is something like this I'm going to have animal question mark animal dot legs colon and then probably something like zero now what this is saying is if animal dot legs is defined so pretty much if it's not undefined right that's kind of what I'm checking here if we want it to be more explicit we could say does not equal undefined then we will use animal dot legs otherwise we will use zero so this is a safe way for us to access that property just wanted to quickly show you them okay so now that we've kind of looked at that I just want to show you an example with some more complicated types and how we can kind of Nest types together so let's say that we have maybe something like a farm type okay on this Farm we're probably going to have animals which means we'll likely want to use our existing animal type so obviously we can do that we can specify animal and say that we're going to have an array of different animals now maybe we actually want to have say animal names to objects well what we could do is use some kind of mapping type which again is a more complicated type in typescript that I'll show you so oftentimes you want to have an object where you have some kind of key that is a specific value that maps to a value well the way that you do that is you define an object here inside of square brackets you specify the key so I'm going to have name which is a string and then this is going to map to the type of animal okay so I've said I have an object inside the object I have keys which are the name of type string and then the value associated with them is an animal so that's how you would do this using objects of course you can get more complicated here have nested objects nested types all that kind of stuff but these are kind of the common you know ways that you're probably going to Define different types all right so continuing here I want to show you a few operators you can use to join types together or to essentially allow for different types so let's say that for some reason maybe we want colors to be equal to a string array or a string we have the ability to do that in typescript by using this operator right here which is the pipe so what I can do now is specify that I have colors and that can be the type of a string array or just a single string I could then of course continue and add different types here as well that this could be equal to now obviously you don't want to go crazy because it makes it a little bit difficult when you're checking these different values and trying to use them but this can be useful if you want to have say two different types or an option for the types now continuing let's say that we have another type and this type is going to have everything that animal has plus a few other values well what I might do is the following let's say we have a type of I don't even know what would make sense here maybe like a monkey and let's say that on our monkey we want everything from animal but we also want to have a diet and let's just say the diet is a string I don't know some description of what they eat well rather than me writing all of this again inside of Monkey what I will do is have this type and then put an and symbol and then put animal now what this will do is an intersection of the two different types which means I must have all the properties on animal and on this original monkey type for it to be type monkey okay hopefully that makes a bit of sense but now if I look at my type monkey it is Diet plus all of the different values from animal so if I wanted to make a monkey I said const m monkey like this now I need to specify all of those types so I'll have my diet which we'll just say is food even though I know that's not very specific and then note here it's giving me an error because I need to specify name age colors Etc all right so just to wrap this up I'm just going to show you a small Edge case here when it comes to annotating types so let's say that we're defining a set for example in JavaScript so const s is equal to new set not setting new set like that and actually that reminds me I'm just going to go to TS config here and I'm going to change es5 to es6 because that's what I need if I want to use a set okay so now set is working fine notice though if I hover over set it says new unknown and then specifies the set type and it has iterable iterable whatever Etc now that's because right now um typescript doesn't know what the type of our set is going to be because we haven't put any values inside of it so what we can do if we wanted to ignore that is we could put at any and now we're not going to have that unknown type and we can just have anything inside of our set however what you're probably going to want to do is specify what the actual type will be so you can say new set and then specify that this is a number inside of these angle brackets and now if I hover over this we know that we have a set that contains numbers we could also do this where we specify the type here so we're going to say new set number like that and now this is the same thing so whatever way you want to do it is fine typically I let it implicitly uh kind of pick up what the type is by just specifying it here but you can obviously annotate it on the variable itself alright so now that we've discussed custom types I want to talk to you about interfaces and the advantage of using them over types in specific scenarios keep in mind this will be a high level overview there is a lot of differences between interfaces and types in typescript I'm just going to scratch the surface here and give you an introduction all right so let's have a look at this example here where we have both a type fish and a type dog now this is fine we have two types they share one property here which is the name and then we have a function that we want to be able to use on both our dog and our fish now in typescript we know that we can simply just put a pipe operator here and specify all of the different types that we can accept as an argument to this function now in this situation here where we have two different types we know that we can access the shared property of these types because well they both have the same property right so both dog and fish have name which means it doesn't matter what you pass here we're able to access name okay so that's fine we can print out their name now the issue arises here when we have all of a sudden like 20 types 30 types 40 types that we potentially want to accept inside of this function that we all know are going to have this name property well I don't want to constantly keep adding you know that other type the other type the other type because really all I care about is that these types have the property name on them so rather than creating a new type and using that here I'm going to specify something known as an interface so I'm going to say interface animal like this and then we're going to specify that name is a required property now what I will do is I will switch the type annotation from fish or dog to be animal now what that allows me to do is to pass any type of object regardless of its type that implements the animal interface which means it simply needs to have this name property on it that allows me now to access name now I could access any other property that is on this interface and this makes it very easy and kind of more reusable for me because I don't need to constantly keep adding types to the type annotation and if for some reason anything changed in my animal interface then it automatically changes here rather than me maybe mistakingly removing a name from my dog or my fish and then breaking all of this code hopefully that makes a tiny bit of sense but typically you're using an interface when you want to view a specific object or type through the lens of another type so the interface is not going to be the type of any individual variable that you define I'm not going to say something like const X animal is equal to and then specify a name I can do that it's valid for me to do that but instead I'm most likely going to have a fish where then I Implement a function like swim so we say swim does this and then I will pass my fish and view it as if it's an animal inside of this function I know that might be a little bit confusing again there's a lot of overlap between how you can use interfaces and types inside of typescript typically though again you're using an interface when you want to ensure that whatever's being passed to a specific function or used in a certain area has certain properties on it but you don't care about the additional properties that exist on that object or what the original type actually is you just want to view it as this specific type alright so now I want to talk about enums or enumeration now to illustrate why we would want to use these let me just paste in a function example here so let's say we have a function where we want to get the price of a specific shirt however the only types of shirts we can have are small medium or large well in this case we can actually just write the three values that we're willing to accept here for shirt size um as the literal strings themselves so small medium large and then maybe we have some kind of switch statement where we're returning you know ten twenty dollars thirty dollars depending on the price of these shirts now that's fine you can do this however a problem arises when you want to change maybe small to be small right now I need to change that here as well and then I need to change any function signatures so that they're now passing that capital S also what happens if I add an additional shirt what happens if maybe I want to use these three values in a different type of function this is not very reusable and this is not great practice so you probably don't want to do something like this instead you'd want to create an enum so what I can do here is say enum shirt size is equal to or not equal to just have my parentheses like this and then I can say small is equal to small medium is equal to medium and large is equal to large and now rather than using small medium and large here I'm simply going to specify that I'm accepting a shirt size which now means I'm going to have one of these three values now for my cases I say shirt size dot small shirt size dot medium and shirt size dot large there you go I've just refactored this code so now first of all it's a little bit more clear what the type actually is and if I go and change any of my string values here like maybe now I want them to be lowercase or I want to add an additional size I can do that and I'm not breaking anything inside of this code now if I wanted to actually use this function what I would do is something like const price if we can type that correctly is equal to get shirt price and then I would pass my shirt size dot small like that so this is just a better practice a better way to do things I'm sure many of you seen enums before these are very useful and a lot of times I like to actually store a bunch of strings inside of my enums you of course can even store something like one two three you can do whatever you want but this allows you again to now use this type in multiple different places rather than writing in literal values which I don't like to be doing unless I have to I'm now using my enumerated value and then again it's kind of more reusable and flexible hopefully you guys can see the benefit of enums now let's move on alright so now I just want to spend a minute discussing what happens if you do actually want to have some kind of function or block of code what you're accepting different types so in this case I have an animal that could be a fish or a dog let's say this is the intended Behavior I actually want to do that and if it's a fish I want to call this swim function if it's a dog then I want to call this bark function well obviously I I can't just come here and go animal dot bark because bark is not a property property sorry that's shared between both the fish and the dog type same with swim I'm gonna get an error if I do that now to get around this what I could do is say animal as fish dot swim and this doesn't give me uh kind of a problem or a red squiggly but this is going to raise a runtime exception If instead of passing a fish I were to pass a dog to this function so before I can run this code with confidence that's actually going to work I need to check what the type of this variable is now unfortunately I can't just use the type of operator that I would normally use that's because our custom types here don't really allow us to use type of now I'll show you what I mean if I try to say if the type of animal is equal to fish which might be what you try you'll notice that this doesn't work it says this condition will always return false since the type's string number begin Etc uh have no overlap so when I check the type of one of my variables here it's only going to return one of these possible values in our case it's going to be an object it's not going to return what the name of the type is that we typed it of typed it as or using typescript that was a lot of types in one sentence anyways how do we get around this well what we actually need to do is create our own custom type check or type guard so what I'm going to do is create a function here called is fish now four is fish I'm gonna take an animal and this animal again could be a fish or a dog and then I'm gonna return from this function the animal is if we can type this correctly fish and then I'm going to have an arrow function I need to return this now what I'm essentially saying when I write animal is fish is that I'm returning if the parameter animal is of type fish so that's what this type annotation means when I write that now inside of here for me to actually check that I need to make sure that a fish has this swim method on it so to do that I'm going to return my animal as a fish dot swim does not equal undefined when I do that notice everything is fine because what I'm doing is I'm first converting my animal to the type fish I'm trying to access the swim property and if that's not undefined that means all is good I can go ahead and I can return my fish or I can return true that this is a fish if swim is undefined then I'm going to return false and that would mean that I had a dog hopefully this makes a little bit of sense I know it's kind of complicated but this is known as a custom guard or a type guard and now we can use this is fish function inside of here to ensure that when we call the fish.swim method it's going to be valid and not give us a runtime exception so I can now say if is fish and this is animal then I can say animal dot swim like that otherwise I can say animal dot buck and notice that now bark is going to work because if it's not a fish then it must be a dog so it's able to kind of imply that using this if else check statement that I've done here now my function is valid just wanted to show you that because sometimes you will need to write this kind of code all right so that is about to wrap up this video the very last thing I want to show you is how you ignore type checking for specific lines sometimes it can be very complicated to actually type annotate something or you're bringing something in from a library and you just want to ignore the kind of squiggly line that you're getting or the problem that's occurring so let's do a very simple example where we have something like const or we say something like let X number equal three and then we say or sorry equal to and then X is equal to hello let's say that we just want to ignore this problem for some reason we do actually want to do this well what I can do is go slash slash at t s Dash ignore and I put that right above this line and that's going to ignore what's on the next line so that I no longer get this compiler error or this what you call it linter error there's all kinds of other kind of comments you can do here that will ignore specific types of problems you're not just ignoring the entire line but I just wanted to show you this shortcut because sometimes it can be useful obviously don't overuse it you shouldn't have to use this but sometimes it is necessary so that's why I wanted to throw it out here anyways with that said I think I'm going to wrap up the video here this was meant to be a quick overview of typescript I think I got a little bit carried away showing you a ton of different features obviously I want to be thorough I want to make sure you guys have enough knowledge to actually be able to use this effectively so if you appreciate shaded the video make sure you leave a like subscribe to the channel and I will see you in the next one